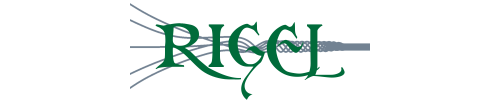
Genetic parameters flags
Macro RIGEL_PARAM_SORTDESC
RIGEL_PARAM_SORTDESC
Set the optimization model to minimization (using population sorting in descending order).
| Note: |
| | By defaults, RIGEL maximizes evaluations. Setting this flag makes RIGEL minimize evaluations. |
Macro RIGEL_PARAM_SELRANKG
RIGEL_PARAM_SELRANKG
Uses ranking as parent selection method.
Macro RIGEL_PARAM_SELTOURN
RIGEL_PARAM_SELTOURN
Uses tournament as parent selection method.
Macro RIGEL_PARAM_SELECTOR
RIGEL_PARAM_SELECTOR
Parent selction method mask.
| Note: |
| | This is equal to RIGEL_PARAM_SELPROPN|RIGEL_PARAM_SELRANKG|RIGEL_PARAM_SELTOURN. |
Macro RIGEL_PARAM_REPRANKG
RIGEL_PARAM_REPRANKG
Uses ranking as offspring replacement method.
Macro RIGEL_PARAM_REPTOURN
RIGEL_PARAM_REPTOURN
Uses tournament as offspring replacement method.
Macro RIGEL_PARAM_REPLACMT
RIGEL_PARAM_REPLACMT
Offspring replacement method mask.
| Note: |
| | This is equal to RIGEL_PARAM_REPPROPN|RIGEL_PARAM_REPRANKG|RIGEL_PARAM_REPTOURN. |
Macro RIGEL_PARAM_REPCOMMA
RIGEL_PARAM_REPCOMMA
Uses comma selection for offsprings (exclude elite).
Macro RIGEL_PARAM_STRATEGY
RIGEL_PARAM_STRATEGY
Selection and replacement strategies mask.
| Note: |
| | This is equal to RIGEL_PARAM_SELCOMMA|RIGEL_PARAM_REPCOMMA. |
Parameters store memory management
Function RIGEL_ParametersAlloc
RIGEL_Parameters RIGEL_ParametersAlloc() ;
Alllocates a new RIGEL_Parameters structure.
| Returns: |
| | _ NULL | : if no memory was available |
| | else . |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | This constructor initializes all parameters with a zero value. |
Function RIGEL_ParametersCopy
RIGEL_Parameters RIGEL_ParametersCopy(RIGEL_Parameters param) ;
Copies a RIGEL_Parameters structure, with its contents.
| Args: |
| | _ param | : parameters set to copy |
| Returns: |
| | _ NULL | : if no memory was available |
| | else a complete copy of the 'param' argument. |
| Errors: |
| | _ ENOMEM | : if no memory was available |
Function RIGEL_ParametersFree
void RIGEL_ParametersFree(RIGEL_Parameters param) ;
Frees a RIGEL_Parameters structure.
| Args: |
| | _ param | : parameters set to free |
Access to hash-stored parameters
Function RIGEL_ParametersGetHash
double RIGEL_ParametersGetHash(RIGEL_Parameters param, char * name) ;
Get hash-stored parameters from a RIGEL_Parameters structure.
| Args: |
| | _ param | : parameter set to query |
| | _ name | : hash key for the parameter |
| Returns: |
| | _ -1 | : if an error occured |
| | else the (positive) parameter value. |
| Errors: |
| | _ EINVAL | : if the parameter set or the hash key were NULL |
Function RIGEL_ParametersSetHash
double RIGEL_ParametersSetHash(RIGEL_Parameters param, char * name, double value) ;
Set hash-stored parameters in a RIGEL_Parameters structure.
| Args: |
| | _ param | : parameter set to update |
| | _ name | : hash key for the parameter |
| | _ value | : a positive value to store |
| Returns: |
| | _ -1 | : if an error occured |
| | else the 'value' argument. |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| | _ EINVAL | : if an argument was invalid |
| Note: |
| | Only positive 'value' arguments are accepted. If the hash entry for 'name' does not exist, it will be created. |
Function RIGEL_ParametersDelHash
double RIGEL_ParametersDelHash(RIGEL_Parameters param, char * name) ;
Delete hash-stored parameters from a RIGEL_Parameters structure.
| Args: |
| | _ param | : parameter set to update |
| | _ name | : hash key for the parameter |
| Returns: |
| | _ -1 | : if an error occured |
| | else . |
| Errors: |
| | _ EINVAL | : if an argument was invalid |
| Note: |
| | Attempting to delete a non-existing hash entry will result in a -1 result, but will not set 'errno'. |
Initialisation and string-based setting of parameters
Function RIGEL_ParametersSetDefaults
RIGEL_Parameters RIGEL_ParametersSetDefaults(RIGEL_Parameters param) ;
Set default parameters in a RIGEL_Parameters structure.
| Args: |
| | _ param | : parameter set to update (if any) |
| Returns: |
| | _ NULL | : if no memory was available |
| | else the updated or allocated parameter set. |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | If 'param' is null, a new parameter set will be allocated. Any hash-stored parameter in the original 'param' argument is unaffected. |
Function RIGEL_ParametersGetDouble
double RIGEL_ParametersGetDouble(RIGEL_Parameters param, char * name) ;
Get a named parameter in a RIGEL_Parameters structure, as a double value.
| Args: |
| | _ param | : parameter set to query |
| | _ name | : parameter name |
| Returns: |
| | _ -1 | : if an argument was invalid |
| | else the parameter value. |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | Non-double parameters (integer and flags) will be converted to double. Casting back to the proper type should result in a correct value, however. |
Function RIGEL_ParametersGetString
char * RIGEL_ParametersGetString(RIGEL_Parameters param, char * name) ;
Get a named parameter in a RIGEL_Parameters structure, as a character string.
| Args: |
| | _ param | : parameter set to query |
| | _ name | : parameter name |
| Returns: |
| | _ NULL | : if an argument was invalid |
| | else a malloc-ed character string representation of the parameter value. |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | The flag parameter will be converted to a human-readable flag representation. |
Function RIGEL_ParametersSet
unsigned long RIGEL_ParametersSet(RIGEL_Parameters param, char * name, char * val) ;
Set a named parameter in a RIGEL_Parameters structure.
| Args: |
| | _ param | : parameter set to update |
| | _ name | : parameter name |
| | _ val | : parameter value string |
| Returns: |
| | _ -1 | : if an argument was invalid |
| | _ 0 | : if a value was not in the correct range |
| | else the original value before the update |
| Errors: |
| | _ EINVAL | : if an argument was invalid |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | The value string will be converted to the actual storage type for this parameter. Any unrecognized built-in parameter name will be stored in hash, provided a parameter with this name has already been defined using RIGEL_ParametersSetHash. |
Argument parsing
Function RIGEL_ParametersParse
void RIGEL_ParametersParse(RIGEL_Parameters param, ...) ;
Parse and stores a NULL-terminated array of couples of parameter name/value.
| Args: |
| | _ param | : parmeter set to update |
| Errors: |
| | _ ENOMEM | : if no memory was available |
Function RIGEL_ParametersParseArgs
void RIGEL_ParametersParseArgs(RIGEL_Parameters param, int * argc, char * * * argv) ;
Parse, store and remove from the argument array '+'-prefixed parameters.
| Args: |
| | _ param |
| | _ argc |
| | _ argv |
| Errors: |
| | _ ENOMEM | : if no memory was available |
Function RIGEL_ReadParameters
unsigned long RIGEL_ReadParameters(RIGEL_Parameters param, int fd) ;
Reads and stores parameters lines from a file.
| Returns: |
| | _ NULL | : if |
| | else . |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | Parameters lines are of the form "name = value", where 'name' is the first word (contiguous serie of non-whitespace charaters) on the line before the '=', and 'value' starts at the first non-whitespace character after the '=' until the end of the line. |
Function RIGEL_WriteParameters
unsigned long RIGEL_WriteParameters(RIGEL_Parameters param, int fd) ;
Writes parameters lines to a file.
| Returns: |
| | _ NULL | : if |
| | else . |
| Errors: |
| | _ ENOMEM | : if no memory was available |
| Note: |
| | Parameters lines are of the form "name = value". |
Generated by textdoc2html - 2006-03-04